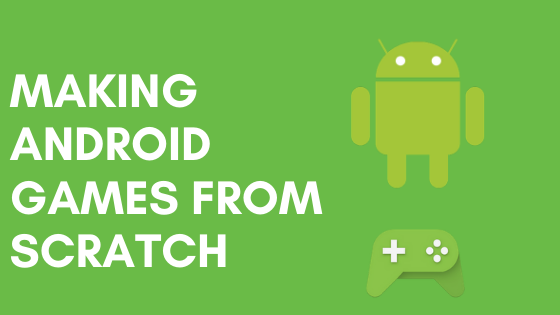
There are two ways you can build something:
1, Build on top of an established system
2, Build from scratch
While building on top is an obvious practice for software that sells, It is when projects are build from scratch which gives you a deeper understanding.
I have spent the last 3 years on and off building games from scratch ( using canvas, media player, bitmaps, threads, classes etc) for Android and here are my experiences:
A lot of times, problems seem easy when you do not understand them properly. Building games involves coming across dozen of such cases on a regular basis where your assessment mismatches reality which is often disappointing. For me, this involved around 80% of issues dealing with threads. You see, when you see a sprite moving on screen independent of game environment, that animation is a thread. A lot of times I used to find myself back at the drawing table trying to add one more level of interaction between the sprite thread and the main game thread.
So before you go ez-pz on a problem, ensure you are not pushing yourself into muddy waters.
This covers everything including documentation for android, an audio library that you are using and surprisingly your very own project. When you are dealing with a lot of parallel ideas, just commenting the code is not enough. Having a running document while you are building the game is the most mundane yet the most efficient of decisions. Your future self or your peers will thank you for this.
You must have come across the statement: "If it's on the internet, its there forever". Sounds good, but there's another side to it. The web is constantly changing. This means while a lot of content is coming into new existence, a fraction of it also snaps out of it never to be seen again. This largely involves mid-tier knowledge that ties low level details with high-level abstract implementations ( looking at you bitmaps). Documentation again can save the day by bundling this knowledge into the project.
Another example is volatility of 3rd party dependencies. Remember Parse by facebook? or TinyPic? No right? They shut down! So no matter what company is behind a service (It was facebook godamnit!), It can disappear. However staying updated with your inbox saves you the hassle of cases like LOOSING ALL DOCUMENTATION DIAGRAMS TO TINYPIC SHUTDOWN! You get the idea what I am implying here.
So your game is good right? You sure buddy? You know how all parents think their newborn is the cutest of all the newborns that have ever existed?!! ( I mean come on peeps! Clearly my future kid is the cutest!).
You will be tempted to ask others to play your game and they might not like it. Don't let this get to your head and above all, never take it personally. I have experienced that giving critical review is one of the most caring acts. What blocks us from reaping the benefit is how we take it. Think for yourself: If you didn't care for the project, why would you tell me what is wrong with it? Thinking this way requires major perception shift, The benefits break major mental bounds for everything you do going ahead.
No Seriously! Its a game after all right? And while you might not have an audience in sight for your project when you begin, you can always pivot to monetise! The struggle you did while building gets fulfilled by the joy when someone appreciates your work. A lot of top titles started out as side hobby projects and they were definitely not the author's first project.
------------------------------------------------------------------------------------------------
If you liked or disliked what you just read, let me know in the comments.
P.s. I do coffee if you pay.
1, Build on top of an established system
2, Build from scratch
While building on top is an obvious practice for software that sells, It is when projects are build from scratch which gives you a deeper understanding.
I have spent the last 3 years on and off building games from scratch ( using canvas, media player, bitmaps, threads, classes etc) for Android and here are my experiences:
1, The seemingly easiest problems are the toughest one:
So before you go ez-pz on a problem, ensure you are not pushing yourself into muddy waters.
2, The documents are never complete:
3, Things on the internet disappear:
Another example is volatility of 3rd party dependencies. Remember Parse by facebook? or TinyPic? No right? They shut down! So no matter what company is behind a service (It was facebook godamnit!), It can disappear. However staying updated with your inbox saves you the hassle of cases like LOOSING ALL DOCUMENTATION DIAGRAMS TO TINYPIC SHUTDOWN! You get the idea what I am implying here.
4, Don't fool yourself:
The point is, just because you invested a lot of time into it, doesn't mean it will turn out great. (This is not about kids anymore, but who am I to judge?)
Being rationally critical of yourself is one of the first steps to building cool things ahead. So if your game sucks big time, Its time to toss it in the bin! ( or get into fixing it if you are that stubborn).
Being rationally critical of yourself is one of the first steps to building cool things ahead. So if your game sucks big time, Its time to toss it in the bin! ( or get into fixing it if you are that stubborn).
Remember, you are in this to learn. Honouring critical feedback is one of those learnings. This brings us to the next point.
5, Honour Feedback:
6, Enjoy the struggle!
------------------------------------------------------------------------------------------------
If you liked or disliked what you just read, let me know in the comments.
P.s. I do coffee if you pay.